Tableau is a tool that can help us to present data visually. There are many data visualization options that can be used in Tableau.
Not only that, the results of processed data in Tableau can be presented on web pages using embed techniques. For public resources, we can directly present them on other websites by copying the embed code obtained from the dashboard. Meanwhile, resources created in private mode can also be displayed on other web pages, but when someone wants to access them, they must log in first.
But there’s no need to worry. Tableau provides the option for visitors to access embedded resources without needing to log in. The way to do this is by adding a token attribute in JWT format to the embed code.
Create & Activate a Connected Apps
The thing that needs to be prepared before using JWT is create Connected Apps and change the status to enabled. To create it, we need to go to the Tableau dashboard then select the Settings menu. On the Settings page, select the Connected Apps tab.

Fill in the name of the Connected Apps, the project that will be used, and the domain that is permitted to access resources on projects connected via Connected Apps. After the Connected Apps have been successfully created, the next step is to retrieve the Secret ID, Secret Value and Client ID. We will use all three to create a JWT token.
These three data can be obtained via the Connected Apps detail page. You do this by clicking on the name of the Connected Apps that you just created. Next, look at the image below to understand the the three data in PHP variables.
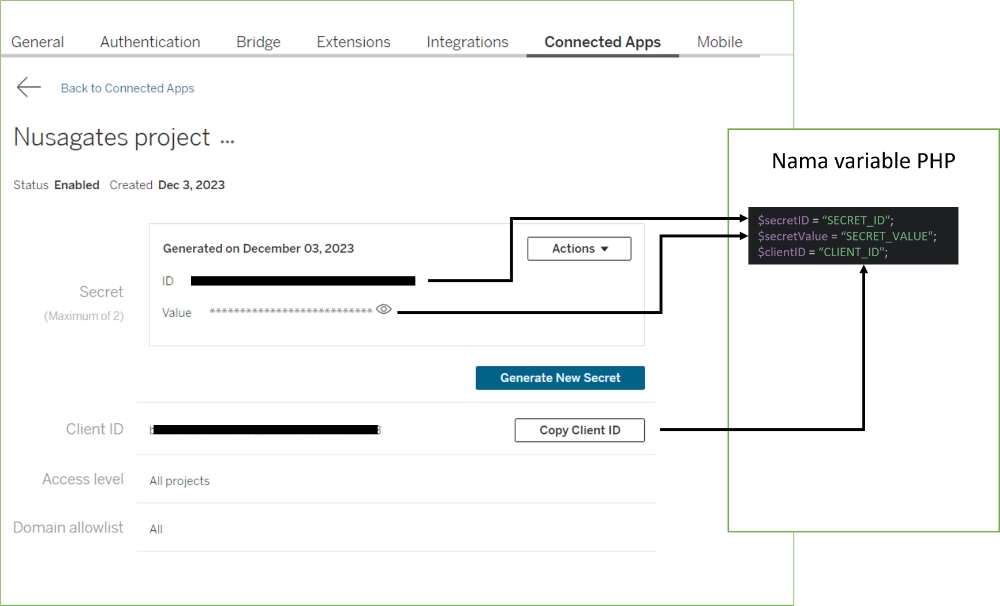
How to Create a JWT Token in PHP
On this occasion, the JWT token will be created using the firebase/php-jwt library which can be installed via composer. How to install it via composer can be seen as below:
composer require firebase/php-jwt
After the firebase/php-jwt dependency is installed, then we can prepare the required data from the Tableau Dashboard.
Create a variable to store the Connected Apps credential data that the Tableau dashboard has retrieved. Look at image 2. These variables are created with the following names:
$secretID = “SECRET_ID";
$secretValue = “SECRET_VALUE";
$clientID = “CLIENT_ID";
As a Tableau documentation, the algorithm supported for creating this JWT is HS256. Declare variables for the algorithm as below:
$algorithm = "HS256";
Create a $headers variable containing kid and iss.
$headers = [
'kid' => $secretID,
'iss' => $clientID
];
Next, create the data payload that will be used as the body.
$issuedAt = new \DateTimeImmutable();
$payload = [
'iss' => $clientID,
"exp" => $issuedAt->modify('+10 minutes')->getTimestamp(),
"jti" => now()->timestamp,
"aud" => "tableau",
"sub" => "USERNAME@TABLEAU",
"scp" => ["tableau:views:embed", "tableau:views:embed_authoring"]
];
Pay attention to the sub section which contains USERNAME@TABLEAU. This section needs to be replaced with the username or email used to log in to the Tableau account.
The scp or scope section contains two values, namely:
- tableau:views:embed to embed data visualization.
- tableau:views:embed_authoring to embed data that can be edited directly by explorer or an account that has editing access.
The last one, we generate JWT using the firebase/php-jwt library with the code below:
return (string) JWT::encode($payload, $secretValue, $algorithm, null, $headers);
The token that has been library generated can then be used to embed Tableau views on the website without asking visitors to log in first. How to embed a Tableau view using this token will be discussed in the next article. If you have questions regarding this discussion, you can contact the author directly via the contact provided in the author box below.